The BME680 looks like a fun little sensor. Lots of values to probe out of one I2C address! I’m trying something a little different with this sensor. I just take soldering for granted. I’ve been doing it for 50 years but I understand that there are people that can’t or won’t OR just don’t have a soldering iron. If you want to play with these sensors WITHOUT soldering, look into the QWIIC connect modules! This system allows you to hook up one (or many) sensor modules without soldering!
Start with the SparkFun Qwiic Shim for Raspberry Pi. It’s a tiny little part that wedges onto the first couple of pins on your Raspberry. You can even put a HAT on over this shim and it doesn’t get in the way! From there you can plug in a (JST PH 4) wire connector that jumpers to the sensor module. For this setup, I’m monitoring a SparkFun Environmental Sensor Breakout. Here’s what the whole setup looks like:
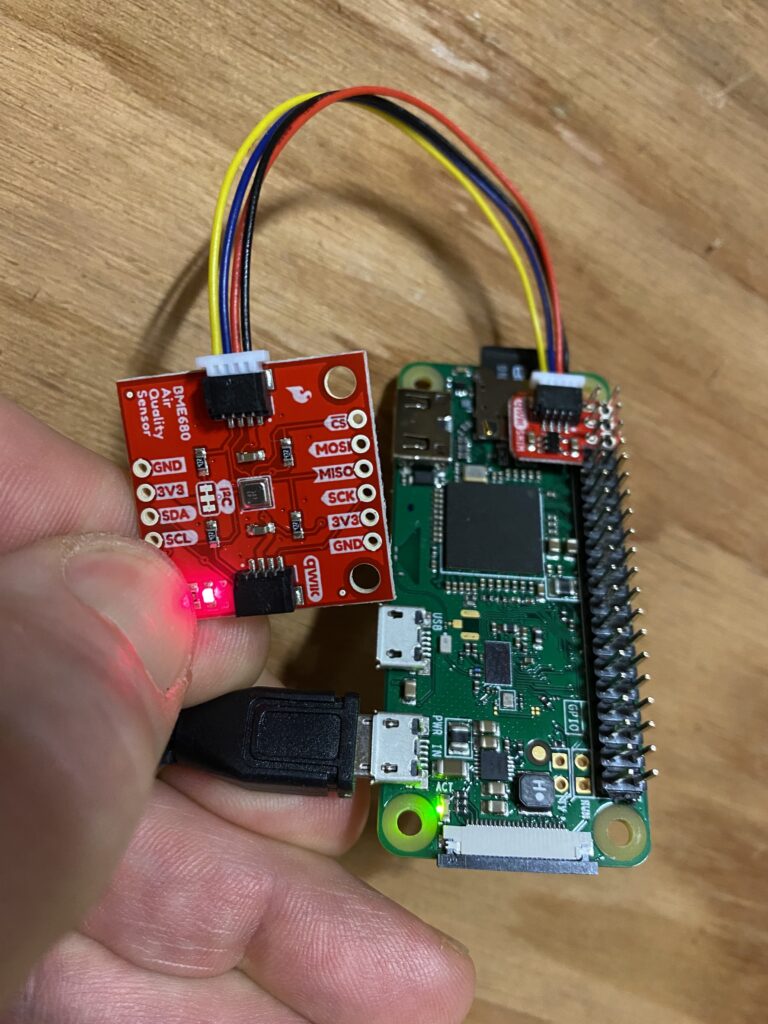
I’ve already installed the CircuitPython programming environment as per this post. Next, I need to install the library for the sensor:
pip3 install adafruit-circuitpython-bme680
Next, the python code example for the BME680. I like to try to find a python3 online to riff from. This one looks like a good candidate.
# SPDX-FileCopyrightText: 2021 ladyada for Adafruit Industries # SPDX-License-Identifier: MIT import time import board import adafruit_bme680 # Create sensor object, communicating over the board's default I2C bus i2c = board.I2C() # uses board.SCL and board.SDA bme680 = adafruit_bme680.Adafruit_BME680_I2C(i2c, debug=False) # change this to match the location's pressure (hPa) at sea level bme680.sea_level_pressure = 1013.25 # You will usually have to add an offset to account for the temperature of # the sensor. This is usually around 5 degrees but varies by use. Use a # separate temperature sensor to calibrate this one. temperature_offset = -5 while True: print("\nTemperature: %0.1f C" % (bme680.temperature + temperature_offset)) print("Gas: %d ohm" % bme680.gas) print("Humidity: %0.1f %%" % bme680.relative_humidity) print("Pressure: %0.3f hPa" % bme680.pressure) print("Altitude = %0.2f meters" % bme680.altitude) time.sleep(1)
Cut and paste this into a file on your Raspberry. There are a couple of modifications to make. Run the program for a couple of minutes to get an idea of the values that it’s giving. We’re going to need to calibrate the temperature and the altitude! The temp is simple. Leave a good thermometer near the sensor and adjust the python script’s “temperature_offset” accordingly.
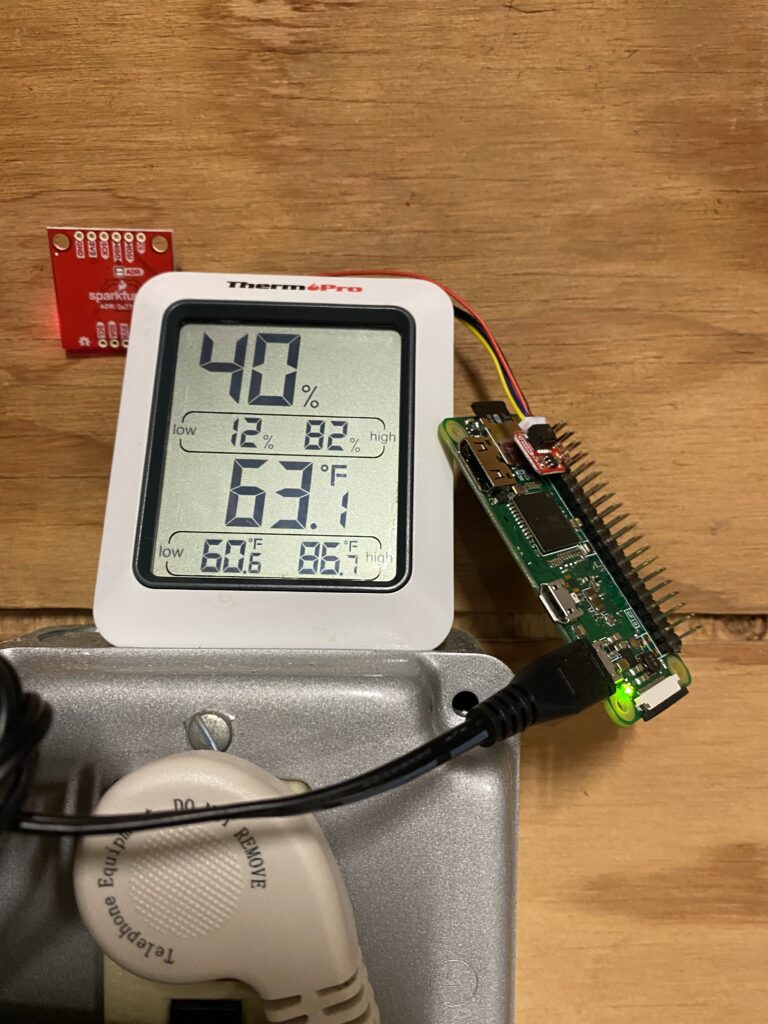
If you prefer Fahrenheit, you can add the conversion to your python3 script:
Celcius = Fahrenheit * 9/5 + 32
If you want to set your altitude in feet, use the conversion:
meters = feet * 3.281
While we’re at it, one last conversion:
inches of mercury = hectopascals * 0.0295
IF you’re clever, you can figure out your current hPa at sea level for your location. Too hard for me. I just tinker with the hPa adjustment until the altitude matches my location. Double-check the barometer setting for your area. Look on Weather Underground and see if one of your neighbors is posting your very local weather. Just click on the temps posted to get the pressure readings for the station. I try to triangulate the pressure at my house based on a couple of local readings around me.
So, after I’m done “calibrating” my readings, here’s what my program looks like:
# SPDX-FileCopyrightText: 2021 ladyada for Adafruit Industries # SPDX-License-Identifier: MIT import time import board import adafruit_bme680 # Create sensor object, communicating over the board's default I2C bus i2c = board.I2C() # uses board.SCL and board.SDA bme680 = adafruit_bme680.Adafruit_BME680_I2C(i2c, debug=False) bme680.sea_level_pressure = 1037.25 temperature_offset = -1.0 barometer_offset = 0.5 humidity_offset = 6 while True: temperature = bme680.temperature + temperature_offset print("\nTemperature: %0.1f F" % (temperature * 9/5 + 32)) print("Gas: %d ohm" % bme680.gas) humidity = bme680.relative_humidity + humidity_offset print("Humidity: %0.1f %%" % humidity ) pressure = bme680.pressure * 0.0295 + barometer_offset print("Pressure: %0.3f inHg " % pressure) feet = bme680.altitude * 3.281 print("Altitude = %0.2f feet" % feet) time.sleep(1)
And the output looks like this:
Temperature: 63.3 F Gas: 45331 ohm Humidity: 39.7 % Pressure: 30.661 inHg Altitude = 398.74 feet